List control
Sourcecode
Please see the UgListControlDemo project for the source code created in this chapter. See Installing examples for information on how to download the examples from https://github.com/scadedoc/UgExamples/tree/master/UgListControlDemo2
Introduction
Our powerful List Control is incredibly versatile, and you can configure it to do many things:
- Its listElement can contain controls of different types
- These control(s) dragged and dropped inside the listElement can also be composed of many other controls
- You can hide and show certain elements programmatically
- You can change a lot of the characteristics by simply binding it to control variables
- The list control uses native graphics as well as platform-specific behaviour, i.e
- Controls within the list control such as label or button are mapped to its respective native iOS and Android platform
- Drag-and-drop, sliding, and other related touch gestures are unique to the iOS and Android platforms and use those platforms' algorithms as well.
Displaying an Array in a List Control
In this example, we want to randomly display 50 European countries with their respective capital.
Add List Control to Page
- We use the constraints box to make sure it uses all the space available
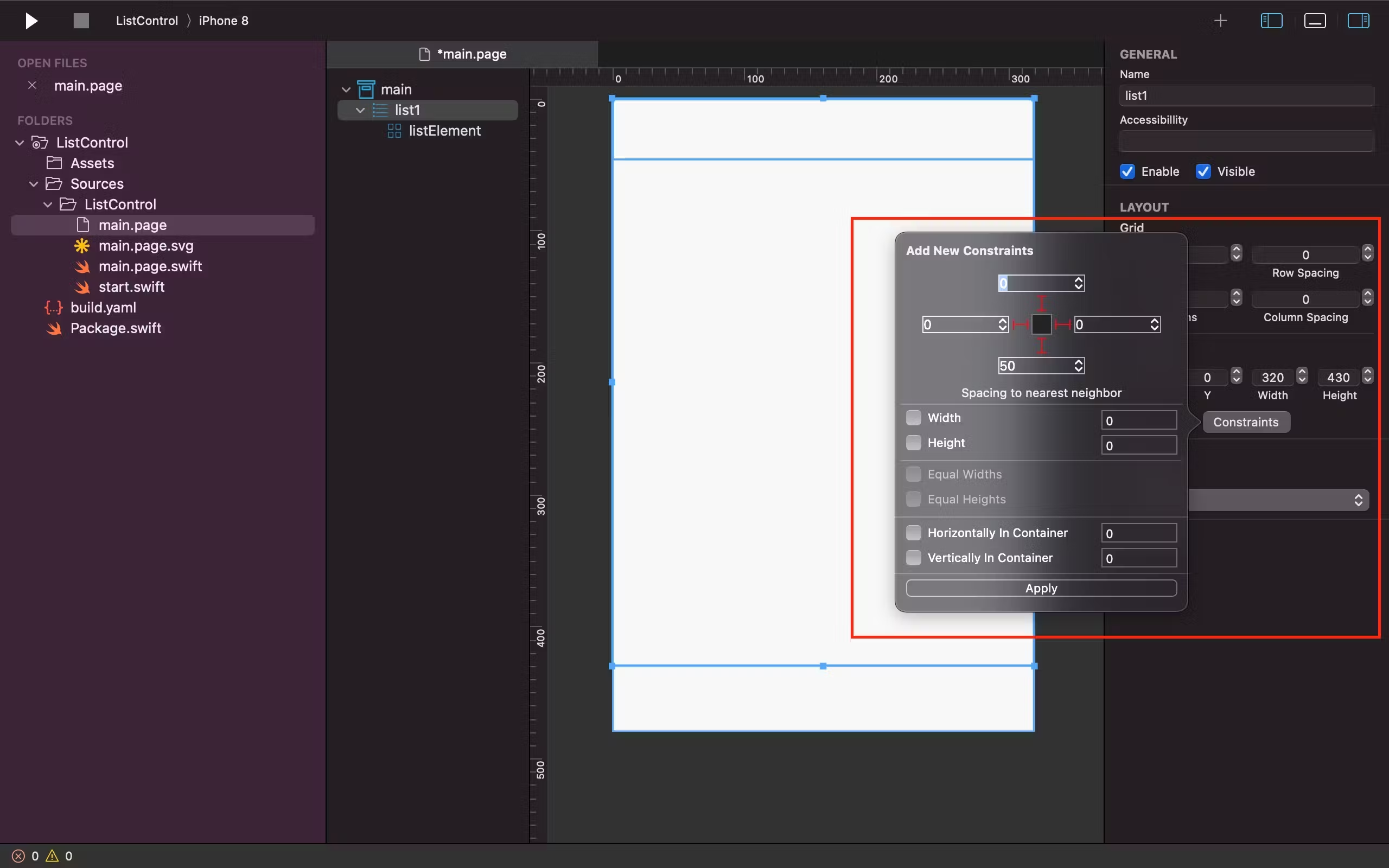
Add controls to listElement
We add the controls we want to see in the listElement
- Each list control contains a list of list elements
- Each list element contains the same visual controls to display the data
- The list element is designed using List Controls's listElement template
- In this example, we added two text labels into a gridView
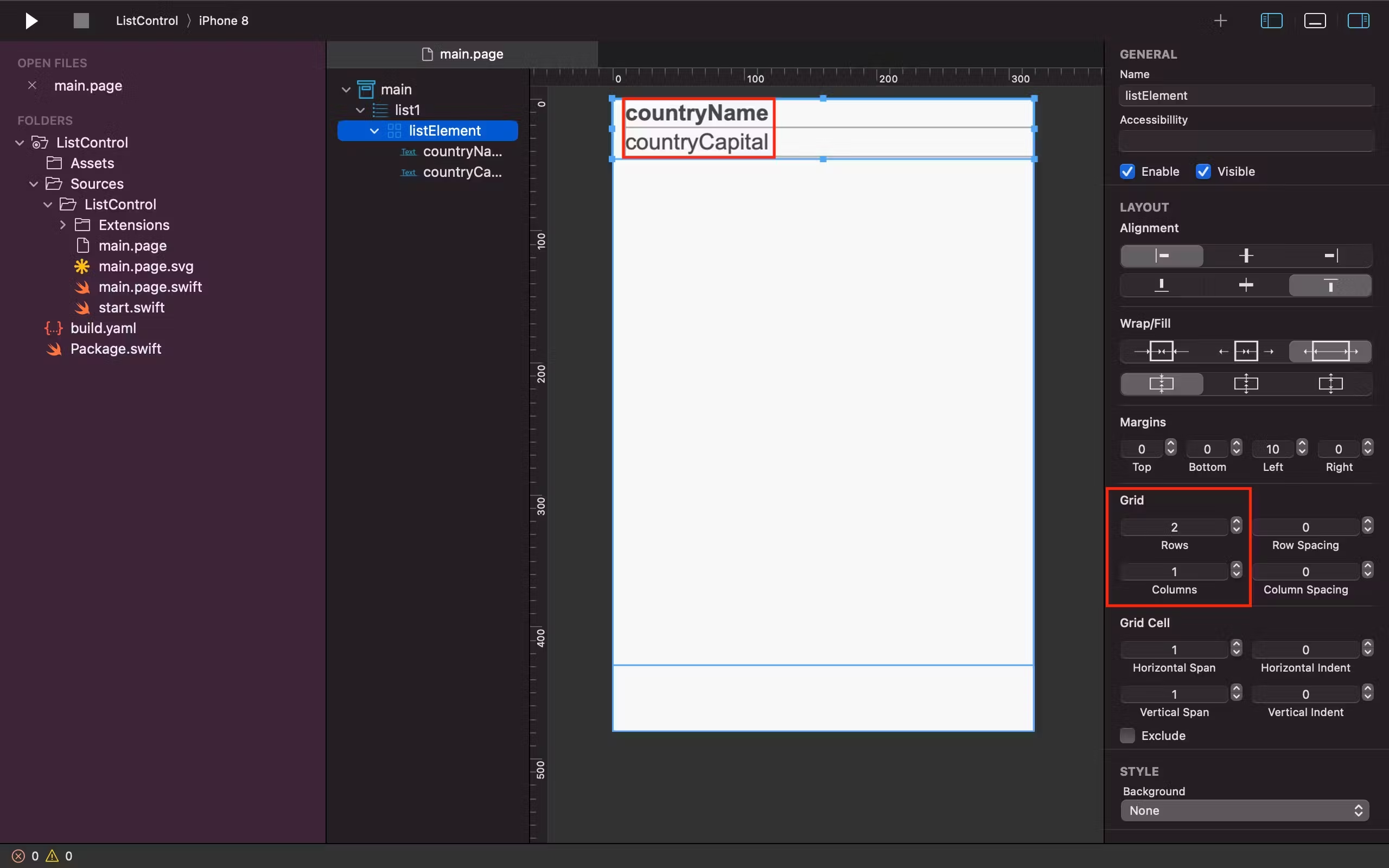
Adding Controls to listElement: Video Illustration
Define Data Model
- We use the Country class to display a list of country names and their respective capitals
- We inherit from
EObject
import ScadeKit
class Country: EObject {
// please make sure you annotate each variable with the type
// for SCADE to more identify and leverage the type information
// currently, you must inherit from EObject
var countryName: String
var countryCapital: String
init(countryName: String, countryCapital: String) {
self.countryName = countryName
self.countryCapital = countryCapital
}
}
Bind data to controls
Bind the list elements visual control to the data
- In order to bind the view to the model, we use the
SCDWidgetsElementProvider
class - Its set using <listControl>.elementProvider
- You set an instance of
SCDWidgetsElementProvider
and add the logic that binds the data to the list element's visual controls - The
template
parameter is of typeSCDWidgetsGridView
. Use thegetWidgetByName
method to retrieve a reference to the visual control and set the data from theCountry
class instance:
class MainPageAdapter: SCDLatticePageAdapter {
var countries: [Country] = []
// page adapter initialization
override func load(_ path: String) {
super.load(path)
self.list1.elementProvider = SCDWidgetsElementProvider { (country: Country, template) in
(template.getWidgetByName("countryName") as! SCDWidgetsLabel).text = country.countryName
(template.getWidgetByName("countryCapital") as! SCDWidgetsLabel).text = country.countryCapital
}
}
}
Set the data source of the control
- Define the source of data by setting the items property
- Its a collection of data objects
list1.items = Array(Countries().countries
List Control lists data successfully
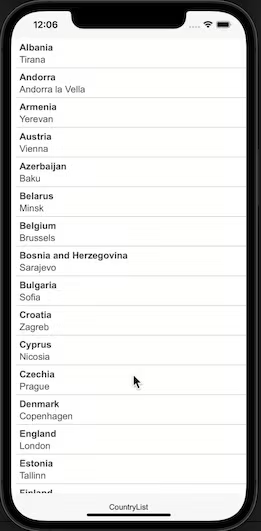
Add handler to process row click / item selected event
To add logic whenever a row is clicked,
- Add the event handler to the .onItemSelected
- The event handler is called SCDWidgetsItemSelectedEventHandler
// the onItemSelected handler is fired when we click a list's row
list1.onItemSelected { event in
if let country = event.item as? Country {
print("Hello \(country.countryName)")
}
}
Create clicked animation effect on list element
This chapter explains how to change the background when a row has been clicked. You need to import both ScadeUI
and ScadeGraphics
to use this awesome feature.
Add the ScadeExtensions repo dependency under the dependencies section of the Package.swift file of your project as it's required for using both ScadeUI
and ScadeGraphics
.
.package(name: "ScadeExtensions", url: "https://github.com/scade-platform/ScadeExtensions", .branch("main"))
We add another handler to the onItemSelected handler array. This time, we use the event's element property to access the list's list element SCDWidgetsListElement and set the animation effect:
- We use gold as a start color
- We use the original color as the end color
- We create an animation effect. We animate the fill property (to fill the background)
- We apply the animation effect to the background of the list element
// add animation when list row is clicked
list1.onItemSelected { event in
guard let element = event.element as? SCDWidgetsListElement,
let backgroundColor = element.backgroundColor
else { return }
// we want to animate the background color from gold to the original color
let fromColor = SCDSvgRGBColor.gold
let toColor = SCDSvgRGBColor(widgetRGB: backgroundColor)
// let us animate fill property
let anim = SCDSvgPropertyAnimation("fill", from: fromColor, to: toColor)
anim.duration = 0.4
anim.repeatCount = 1
anim.delay = 0.2
anim.deleteOnComplete = true
element.backgroundRect?.animations.append(anim)
}
Ensure background is set with fill color
The list element's background can be set to a. Nothing b. Image c. FIll Color
If the background is set to Nothing, no visual component exists and the background cannot be set. Therefore, its mandatory that you set the background to a background color:
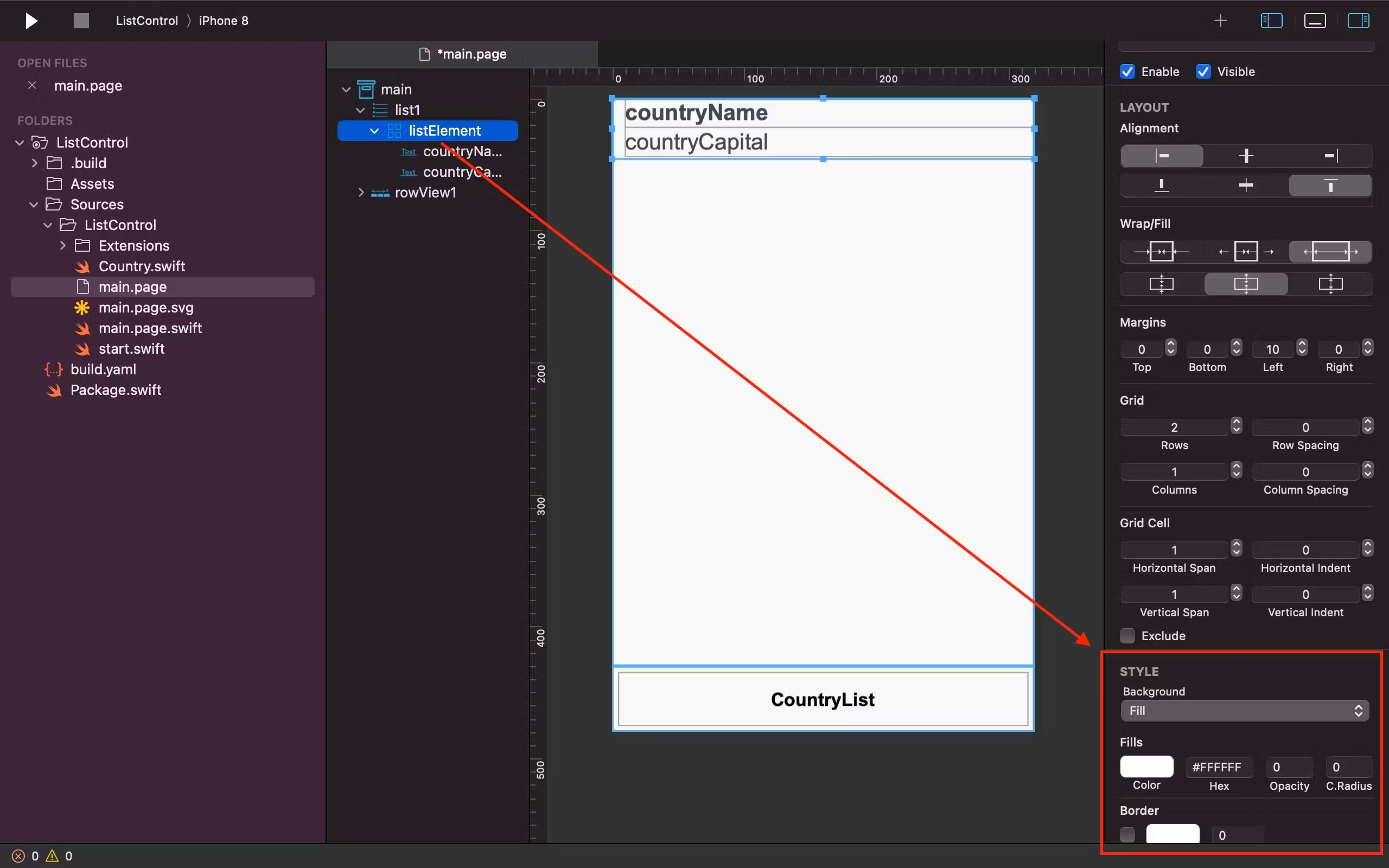
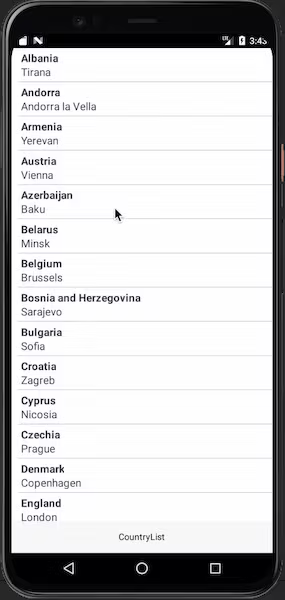
The List Control Video Tutorial
Updated 7 months ago